React State Management Tools: Comprehensive Comparison and Guide Which is better ?
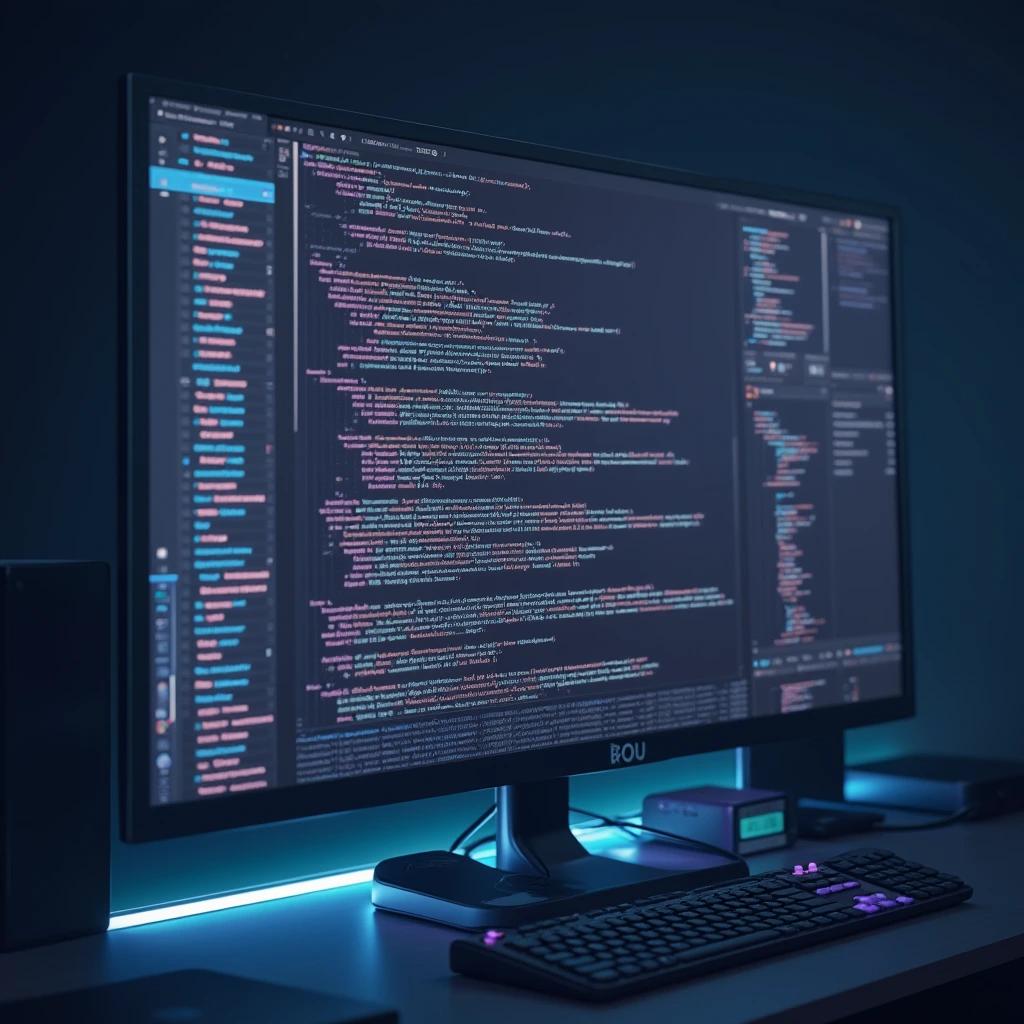
Jan 8, 2025
State Management Tools: Detailed Comparison and Examples
1. Redux
Redux is one of the most popular state management libraries for React applications. It's based on the Flux architecture and uses a single store to manage all the application state.
Key features:
- Centralized store of state
- Time travel debugging
- Middleware support for asynchronous actions
Pros:
- Ideal for large, complex applications
- Excellent developer tools
- Wide ecosystem and community support
Cons:
- Steeper learning curve
- Can be verbose, requires a lot of boilerplate code
- Might be overkill for smaller applications
Best for: Large applications with complex state logic and many moving parts that need centralized state management.
Installation:
npm install redux react-redux
2. MobX
MobX is a simpler alternative to Redux that uses observables to manage and automatically track state changes.
Key features:
- Reactive state management
- Automatic tracking of state changes
Pros:
- Less boilerplate than Redux
- More flexible, less opinionated
- Easy to learn and use
Cons:
- Can be less predictable than Redux in larger applications
- Can be difficult to debug
- Smaller ecosystem than Redux
Best for: Medium-sized applications that require a simpler state management solution and more flexibility.
Installation:
npm install mobx mobx-react-lite
yarn add mobx mobx-react-lite
3. Recoil
Recoil is a new state management library developed by Facebook that aims to solve some of the limitations of Redux and Context API.
Key features:
- Atomic state management
- Derived state using selectors
- Built-in asynchronous state management
Pros:
- Designed specifically for React
- Great performance, especially for large applications
- Easy to share state across components
Cons:
- Still relatively new and evolving
- Smaller community and ecosystem
- Can have frequent breaking changes
Best for: React applications that require fine-grained state management and better performance than the Context API.
Installation:
npm install recoil
yarn add recoil
4. Zustand
Zustand is a small state management solution designed to simplify the complexities typically associated with state management.
Key features:
- Simple API
- No Provider required
- Built-in support for immer
Pros:
- Very lightweight and easy to learn
- TypeScript support is very good
- Works well with React's concurrent mode
Cons:
- Not as well-suited for complex state logic
- Smaller ecosystem and community compared to Redux or MobX
Best for: Applications that need a lightweight and easy-to-use state management solution.
Installation:
npm install zustand
yarn add zustand
5. Jotai
Jotai is another simple state management library that focuses on atomic state management.
Key features:
- Atomic model
- Easy integration with existing React components
Pros:
- Very small package size
- Simple API
- Good for managing form state
Cons:
- Newer library with smaller community
- May lack some features of more mature libraries
Best for: Applications that require lightweight, atomic state management, especially for forms or other granular optimizations.
Installation:
npm install jotai
yarn add jotai
6. Redux Toolkit
Redux Toolkit is an official, opinionated set of tools from the Redux team that aims to simplify the process of working with Redux.
- Includes utilities to simplify Redux setup, including configuration and middleware setup
- Improves developer experience by reducing boilerplate code
- Provides built-in support for creating asynchronous actions with
createAsyncThunk
- Encourages writing "slices" for better organization of state and reducers
Pros:
- Significantly reduces boilerplate code compared to traditional Redux
- Built-in tools for managing asynchronous logic
- Improved developer experience and easier to maintain code
Cons:
- Still requires knowledge of core Redux concepts
- Some additional learning for developers who are already familiar with standard Redux
Best for: Applications that want to use Redux with less boilerplate and better support for asynchronous operations.
Installation:
npm install @reduxjs/toolkit react-redux
yarn add @reduxjs/toolkit react-redux
Considerations for Choosing a State Management Tool
- Application size and complexity: Larger, more complex applications may benefit from a more robust solution like Redux or Redux toolkit, while smaller applications may prefer lighter options like Zustand or Jotai.
- Team familiarity: Consider your team's experience with different state management patterns.
- Performance needs: If you're dealing with frequent, fine-grained updates, a tool like Recoil or Jotai might be a better fit.
- Ecosystem and community support: More mature tools like Redux have larger ecosystems that can help solve common problems.
- Learning curve: Some tools (like Redux) have a steeper learning curve but offer more features and control; others (like Zustand) are easier to get started with but may have limitations.
Note that it's also possible to use multiple state management solutions in a single application, choosing the appropriate tool based on the specific needs of different parts of the state.
Similar Articles
View all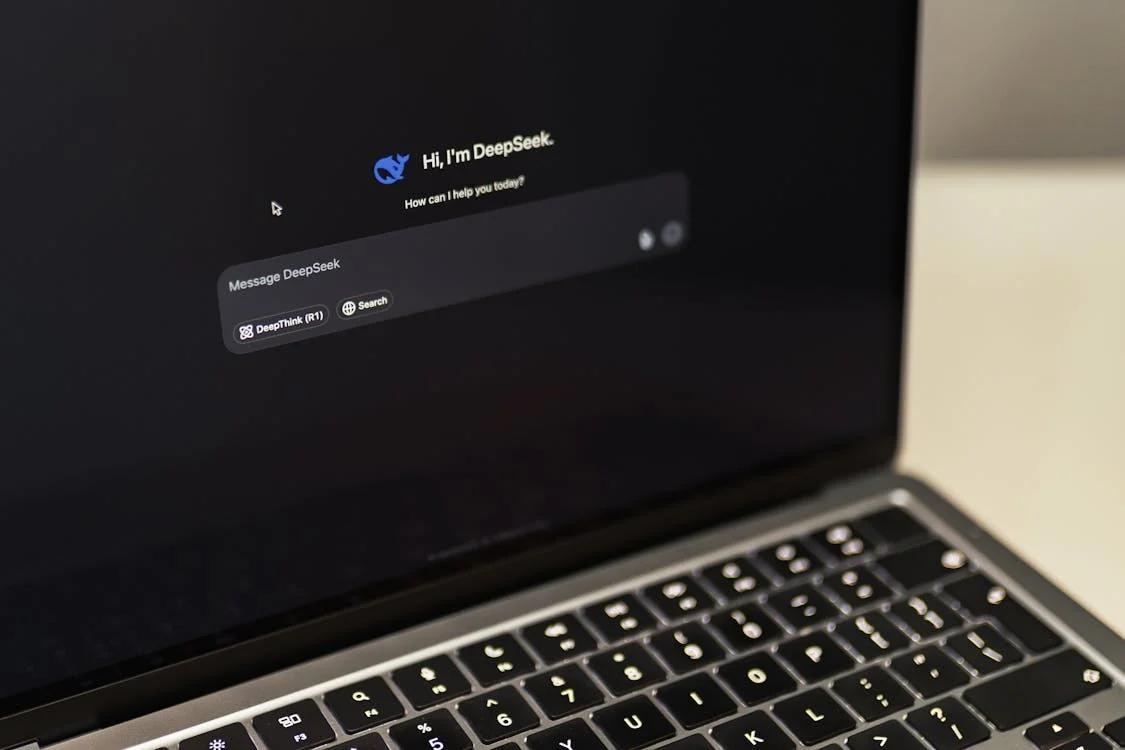
Top 5 AI Technologies Revolutionizing the World in 2025
Find the top AI firms and most impactful AI technologies that will propel innovation in the field in 2025. These cutting-edge AI technologies, which range from Generative AI and Natural Language Processing (NLP) to Computer Vision and Reinforcement Learning, are transforming sectors such as robotics, healthcare, and content production. OpenAI, Google DeepMind, Microsoft, NVIDIA, Deepseek and Tesla playing vital role
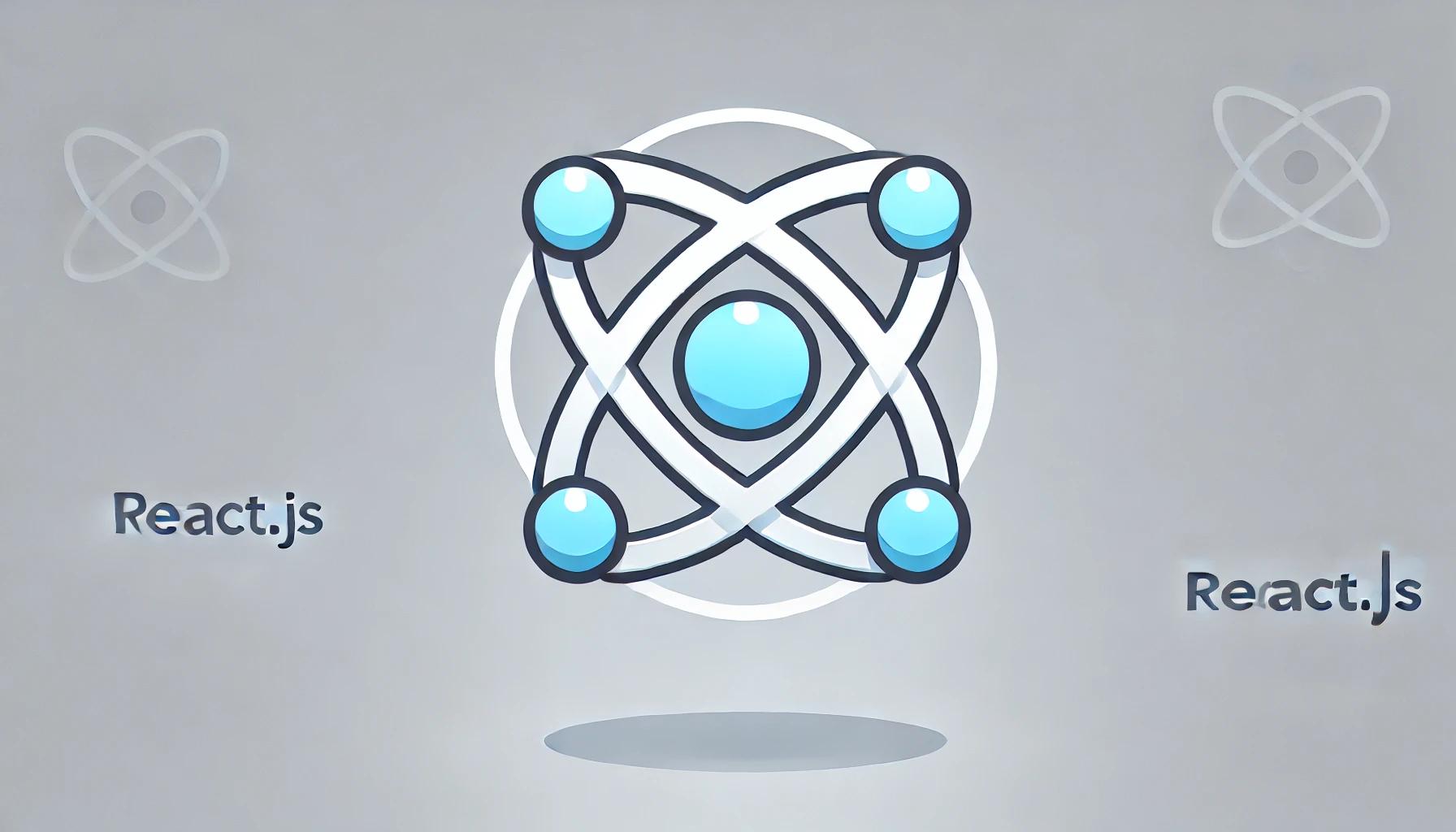
The Must-Know popular JavaScript Frameworks of 2024: A Developer's Friendly article
ive into the exciting world of JavaScript frameworks! Whether you're a coding newbie or a seasoned dev looking to level up, this guide breaks down 2024's hottest frameworks in plain English. From React's powerful ecosystem to Svelte's revolutionary approach, discover which framework fits your coding style and project needs.
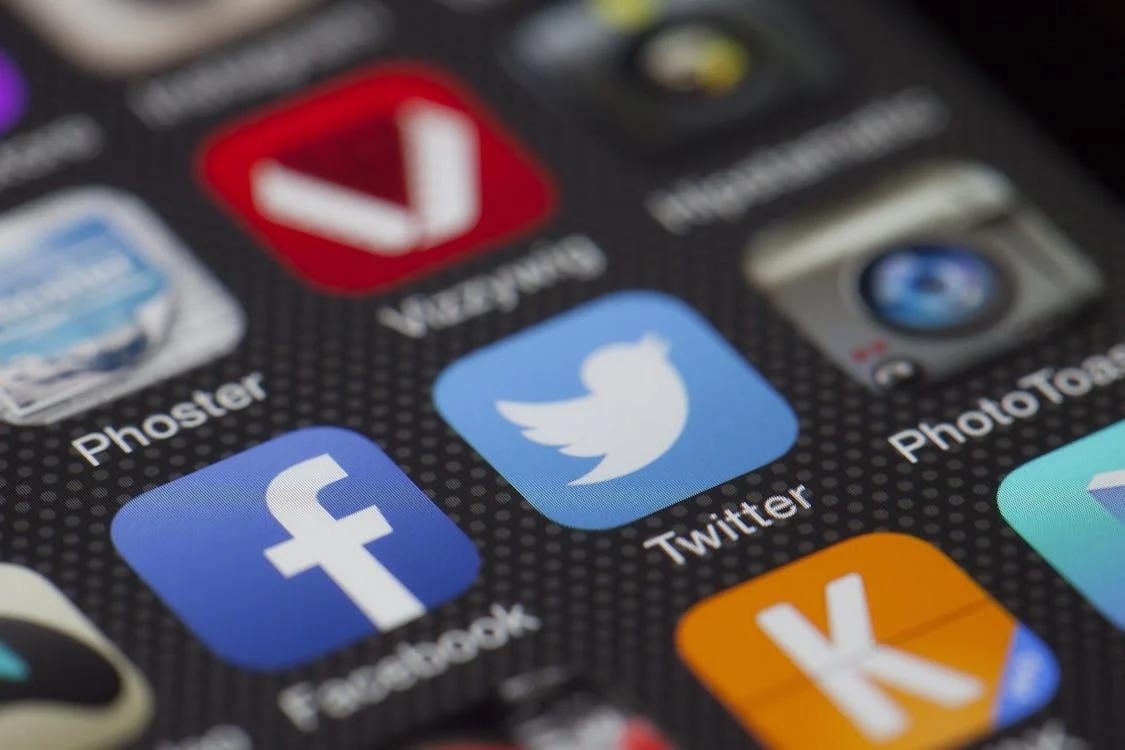
Top cross-platform app development frameworks in 2024
Explore the top cross-platform mobile development frameworks in 2024. Learn about Flutter, React Native, Kotlin Multiplatform, and more to choose the best framework for your projects